- Introduction to MongoDB >
- Getting Started
Getting Started¶
- Mongo Shell
- Compass
- Python
- Node.js
The following tutorial uses the mongo
shell to
insert data and perform query operations.
MongoDB Compass is the GUI for MongoDB. The following tutorial uses MongoDB Compass to connect to insert data and perform query operations.
The following tutorial uses the PyMongo Python driver to insert data and perform query operations.
The following tutorial uses the MongoDB Node.js Driver to insert data and perform query operations.
Prerequisites¶
This tutorial requires you to be connected to one of the following:
- MongoDB Atlas Free Tier Cluster. MongoDB Atlas is a fast, easy, and free way to get started with MongoDB. To learn more, see the Getting Started with Atlas tutorial.
- Local MongoDB installation. For more information on installing MongoDB locally, see Install MongoDB.
Insert Documents¶
- Mongo Shell
- Compass
- Python
- Node.js
db.collection.insertMany()
can insert multiple
documents into a
collection. Pass an array of
documents to the method.
The following example inserts new documents into the inventory
collection:
insertMany()
returns a document that includes
the newly inserted documents _id
field values. See the
reference for an example.
Use db.collection.insertOne()
to insert a single document.
Click Create Database.
Enter “retail” for the Database Name and “inventory” for the Collection Name then click Create Database. For more information, see Databases and Collections.
From the Collections tab, click the “inventory” collection link.
Click the Insert Document button.
Insert the following document by entering the
fields
andvalues
, then selecting the appropriate types from the dropdowns. Add fields by clicking the last line number, then clicking Add Field After ….- For
Object
types, add nested fields by clicking the last field’s number and selecting Add Field After …. - For
Array
types, add additional elements to the array by clicking the last element’s line number and selecting Add Array Element After ….
- For
Repeat steps 4 and 5 to add additional documents:
pymongo.collection.Collection.insert_many()
can insert
multiple documents into a
collection. Pass an array of
documents to the method.
The following example inserts new documents into the inventory
collection:
insert_many()
returns a
document that includes the newly inserted documents _id
field
values. See the reference for an example.
Use pymongo.collection.Collection.insert_one()
to insert a
single document.
Collection.insertMany() can insert multiple documents into a collection. Pass an array of documents to the method.
The following example inserts new documents into the
inventory
collection:
insertMany() returns a
document that includes the newly inserted documents _id
field
values. See the reference for an example.
Use Collection.insertOne() to insert a single document.
For more information and examples, see Insert Documents in the CRUD section.
Query Documents¶
Select All Documents¶
- Mongo Shell
- Compass
- Python
- Node.js
To select all documents in the collection, pass an empty document as the
query filter document to the
db.collection.find()
method:
Select the inventory
collection from the Collections
tab or the left-hand pane. By default, all documents are selected and
additional documents are loaded by scrolling down.
To explicitly select all documents in the collection, pass an empty
query filter document { }
to
the Filter input and click Find.
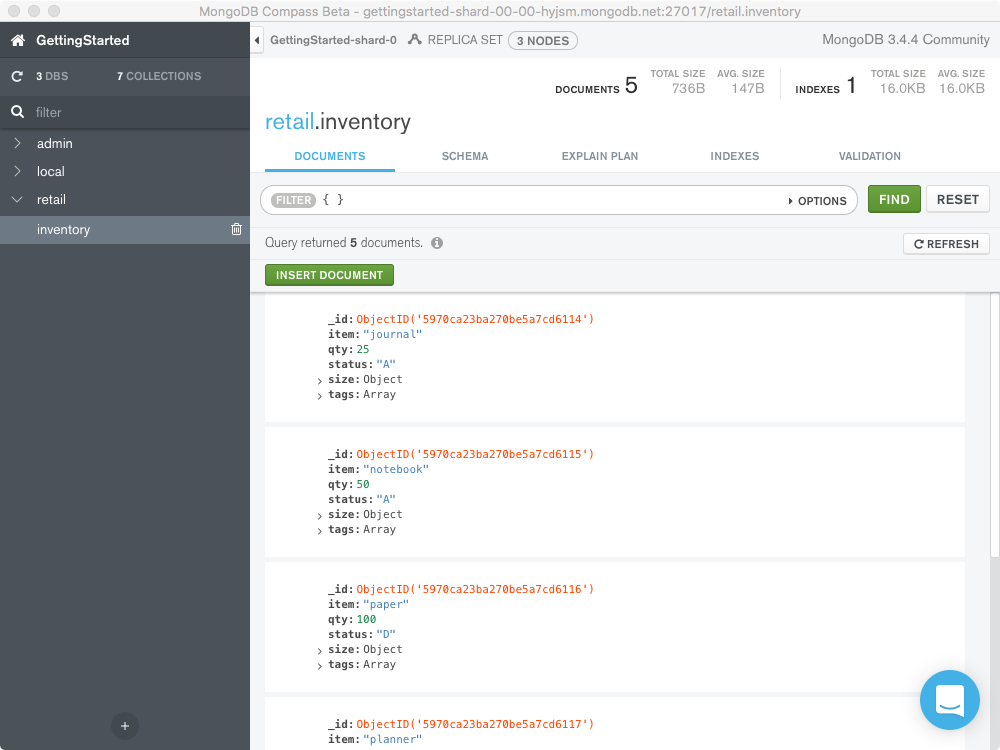
To select all documents in the collection, pass an empty document as the
query filter document to the
pymongo.collection.Collection.find()
method:
To select all documents in the collection, pass an empty document as the query filter document to the Collection.find() method:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
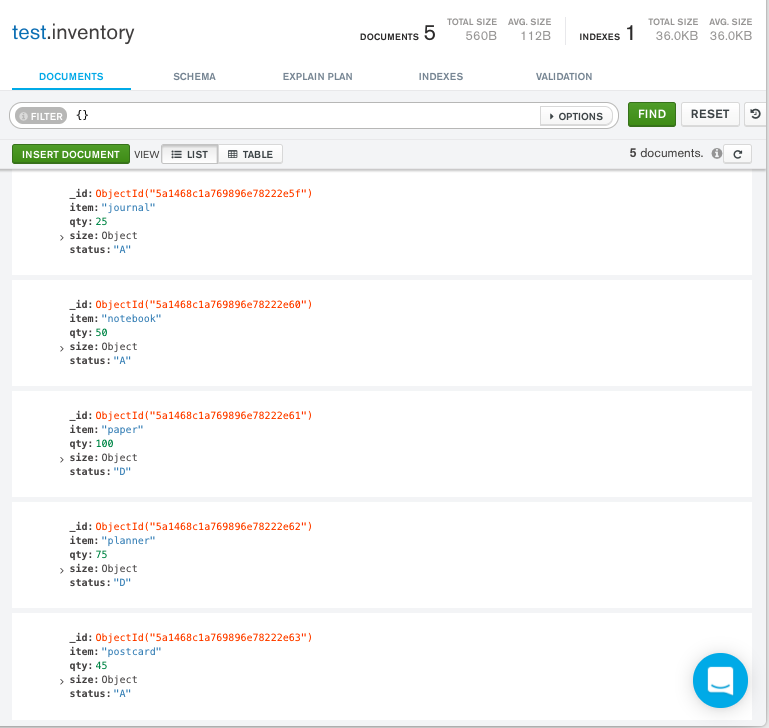
- Mongo Shell
- Compass
- Python
- Node.js
To query for documents that match specific equality conditions, pass the
find()
method a query filter document
with the <field>: <value>
of the desired documents. The following example
selects from the inventory
collection all documents where the status
equals "D"
:
To query for documents that match specific equality conditions, pass
a query filter document to the
Filter with the <field>: <value>
of the desired
documents. The following query filter document selects all documents
where the status
equals "D"
from the inventory
collection:
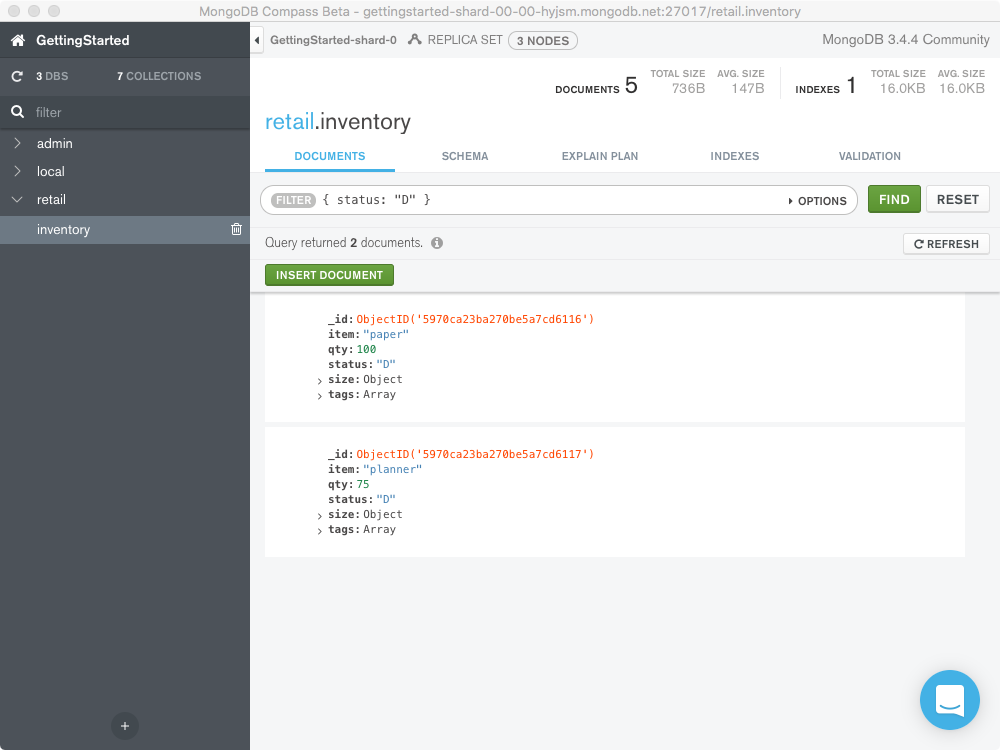
To query for documents that match specific equality conditions, pass the
find()
method a
query filter document with the
<field>: <value>
of the desired documents. The following example
selects from the inventory
collection all documents where the
status
equals "D"
:
To query for documents that match specific equality conditions, pass the
find() method a
query filter document with the
<field>: <value>
of the desired documents. The following example
selects from the inventory
collection all documents where the
status
equals "D"
:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
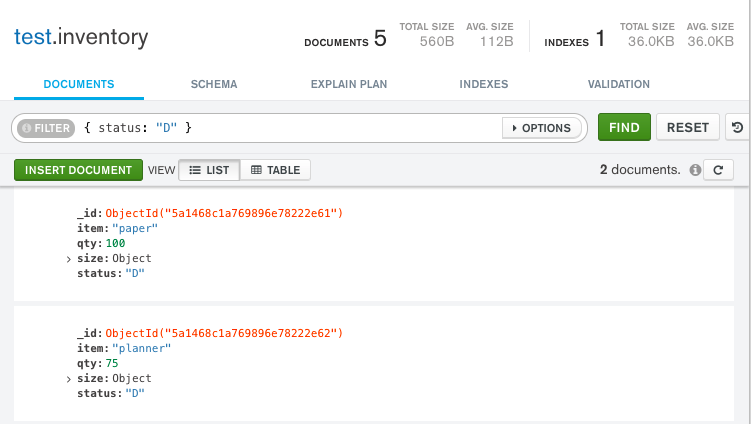
Match an Embedded Document¶
Equality matches on the whole embedded document require an exact
match of the specified <value>
document, including the field order. For
example, the following query selects all documents where the field size
equals the document { h: 14, w: 21, uom: "cm" }
:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
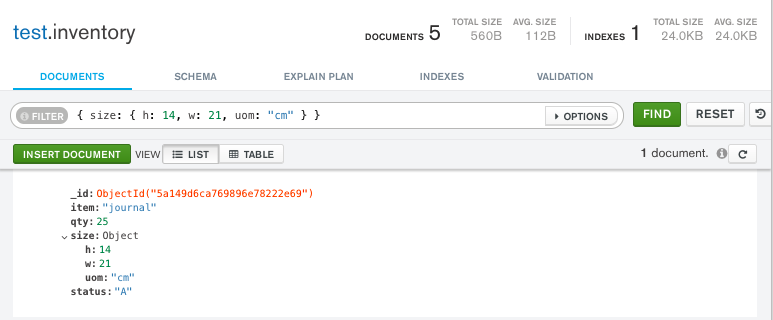
- Compass
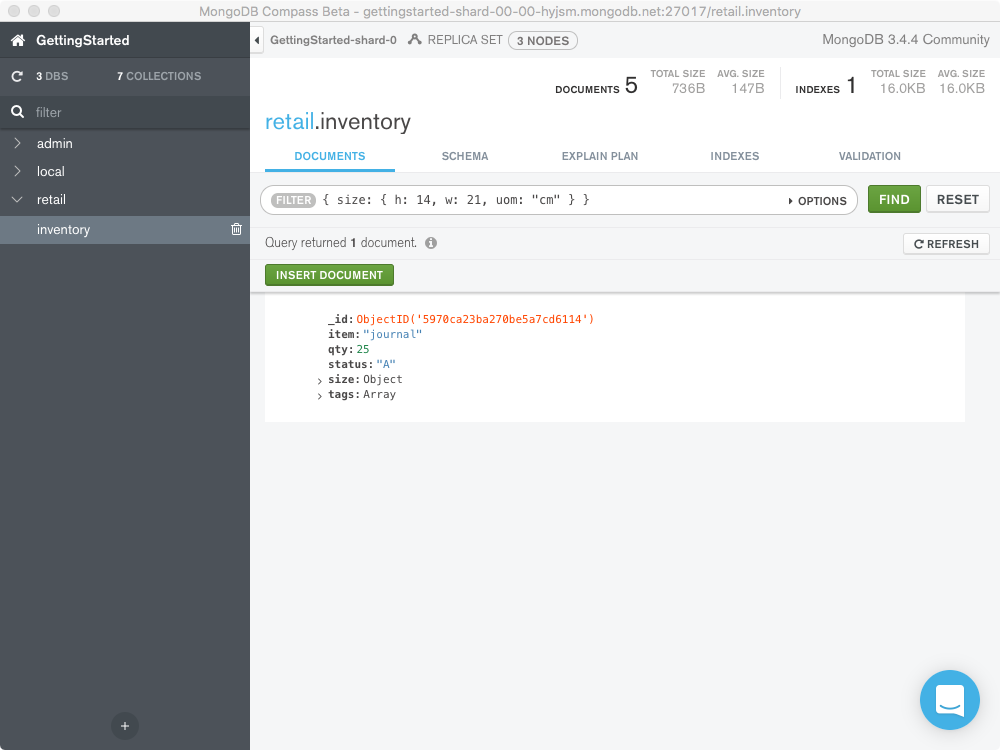
Match a Field in an Embedded Document¶
The following example selects all documents where the field uom
nested in the size
field equals the string value "in"
:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
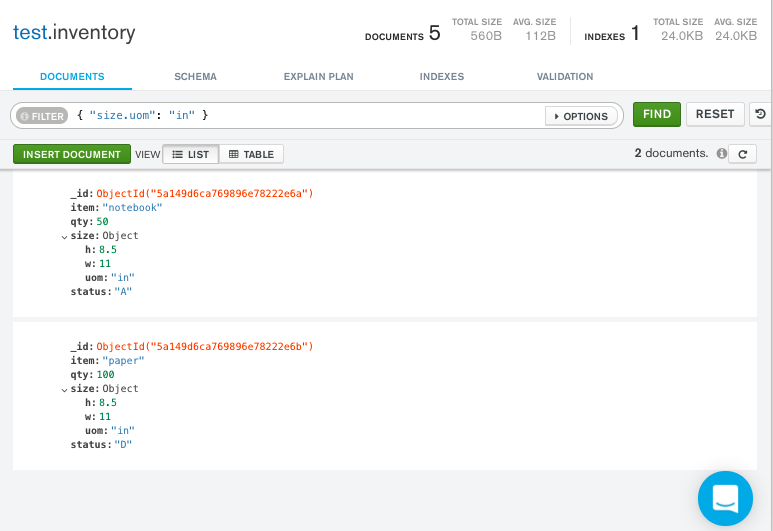
- Compass
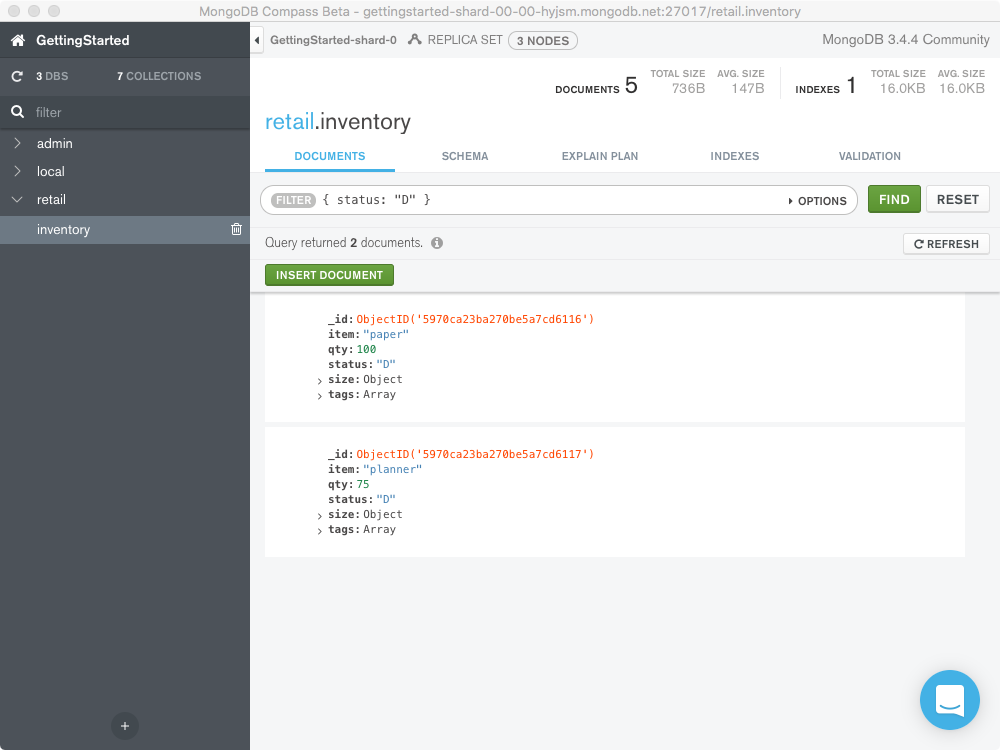
Match an Element in an Array¶
The following example queries for all documents where tags
is an
array that contains the string "red"
as one of its elements:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
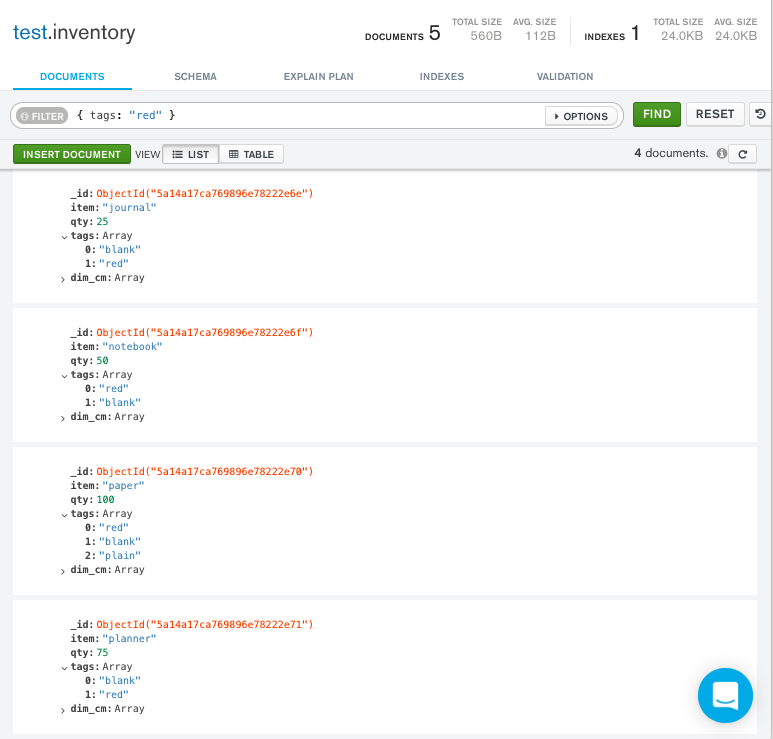
- Compass
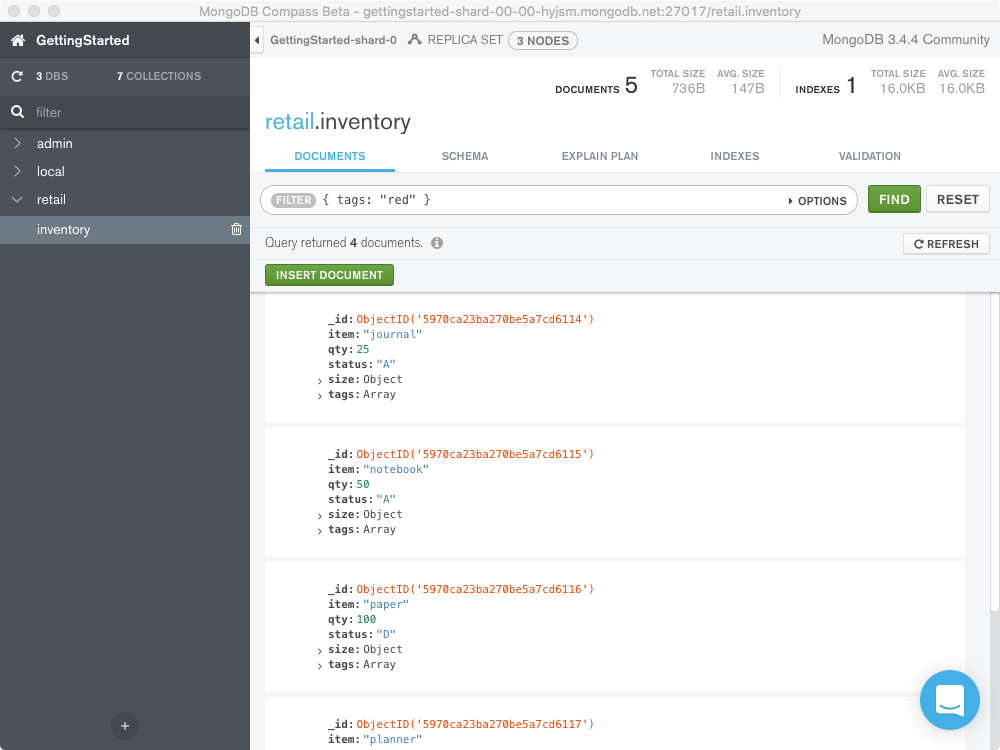
Match an Array Exactly¶
The following example queries for all documents where the field tags
value is an array with exactly two elements, "red"
and "blank"
,
in the specified order:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
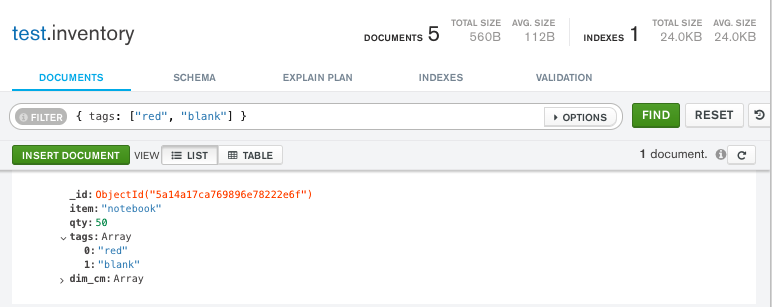
- Compass
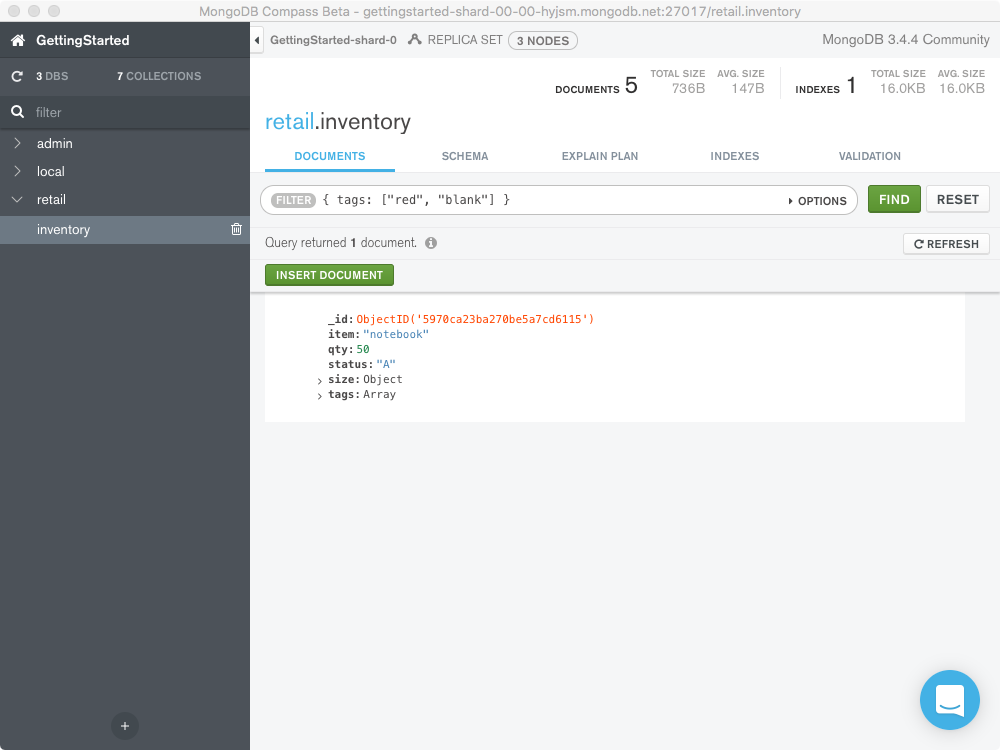
For more information and query examples, see Query Documents in the CRUD section.
To update or delete documents, see Update Documents and Delete Documents.
Next Steps¶
Once you complete the Getting Started Guide, you may find the following course and topics useful:
To learn more about the MongoDB query language and other MongoDB fundamentals, sign up for M001: MongoDB Basics.
Introduction | Developers | Administrators | Reference |
---|---|---|---|