- MongoDB CRUD Operations >
- MongoDB CRUD Concepts >
- Read Operations >
- Read Operations Overview
Read Operations Overview¶
On this page
Read operations, or queries, retrieve data stored in the database. In MongoDB, queries select documents from a single collection.
Queries specify criteria, or conditions, that identify the documents that MongoDB returns to the clients. A query may include a projection that specifies the fields from the matching documents to return. The projection limits the amount of data that MongoDB returns to the client over the network.
Query Interface¶
For query operations, MongoDB provides a db.collection.find()
method. The method accepts both the query criteria and projections and
returns a cursor to the matching documents. You
can optionally modify the query to impose limits, skips, and sort
orders.
The following diagram highlights the components of a MongoDB query operation:

The next diagram shows the same query in SQL:
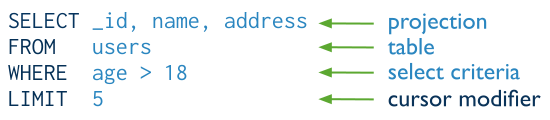
Example
This query selects the documents in the users
collection that
match the condition age
is greater than 18
. To specify the
greater than condition, query criteria uses the greater than (i.e.
$gt
) query selection operator.
The query returns at most 5
matching documents (or more
precisely, a cursor to those documents). The matching documents will
return with only the _id
, name
and address
fields. See
Projections for details.
See
SQL to MongoDB Mapping Chart for additional examples of MongoDB queries and the corresponding SQL statements.
Query Behavior¶
MongoDB queries exhibit the following behavior:
- All queries in MongoDB address a single collection.
- You can modify the query to impose
limits
,skips
, andsort orders
. - The order of documents returned by a query is not defined unless you
specify a
sort()
. - Operations that modify existing documents (i.e. updates) use the same query syntax as queries to select documents to update.
- In aggregation pipeline, the
$match
pipeline stage provides access to MongoDB queries.
MongoDB provides a db.collection.findOne()
method as a
special case of find()
that returns a single
document.
Query Statements¶
Consider the following diagram of the query process that specifies a query criteria and a sort modifier:
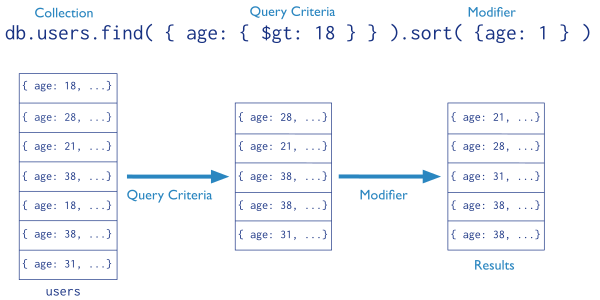
In the diagram, the query selects documents from the users
collection. Using a query selection operator to define the conditions for matching documents,
the query selects documents that have age
greater than (i.e.
$gt
) 18
. Then the sort()
modifier
sorts the results by age
in ascending order.
For additional examples of queries, see Query Documents.
Projections¶
Queries in MongoDB return all fields in all matching documents by default. To limit the amount of data that MongoDB sends to applications, include a projection in the queries. By projecting results with a subset of fields, applications reduce their network overhead and processing requirements.
Projections, which are the the second argument to the
find()
method, may either specify a list of
fields to return or list fields to exclude in the result documents.
Important
Except for excluding the _id
field in inclusive
projections, you cannot mix exclusive and inclusive projections.
Consider the following diagram of the query process that specifies a query criteria and a projection:
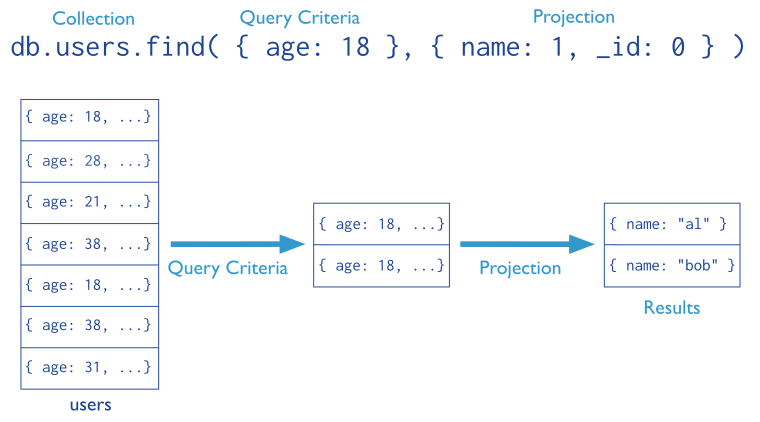
In the diagram, the query selects from the users
collection. The
criteria matches the documents that have age
equal to 18
. Then
the projection specifies that only the name
field should return in
the matching documents.
Projection Examples¶
Exclude One Field From a Result Set¶
This query selects a number of documents in the records
collection that match the query { "user_id": { $lt: 42} }
, but
excludes the history
field.
Return Two fields and the _id
Field¶
This query selects a number of documents in the records
collection
that match the query { "user_id": { $lt: 42} }
, but returns
documents that have the _id
field (implicitly included) as well as
the name
and email
fields.
Return Two Fields and Exclude _id
¶
This query selects a number of documents in the records
collection that match the query { "user_id": { $lt: 42} }
, but
only returns the name
and email
fields.
See
Limit Fields to Return from a Query for more examples of queries with projection statements.
Projection Behavior¶
MongoDB projections have the following properties:
- In MongoDB, the
_id
field is always included in results unless explicitly excluded. - For fields that contain arrays, MongoDB provides the following
projection operators:
$elemMatch
,$slice
,$
. - For related projection functionality in the aggregation
framework pipeline, use the
$project
pipeline stage.