- MongoDB CRUD Operations >
- Query Documents >
- Query an Array of Embedded Documents
Query an Array of Embedded Documents¶
This page provides examples in:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
This page provides examples of query operations on an array of nested documents using the
db.collection.find()
method in mongo
.
The examples on this page use the inventory
collection. Connect to a
test database in your MongoDB instance then create the inventory
collection:
This page provides examples of query operations on an array of nested documents using MongoDB Compass.
The examples on this page use the inventory
collection. Connect to a
test database in your MongoDB instance then create the inventory
collection:
This page provides examples of query operations on an array of nested documents using the
pymongo.collection.Collection.find()
method in the
PyMongo
Python driver.
The examples on this page use the inventory
collection. Connect to a
test database in your MongoDB instance then create the inventory
collection:
This page provides examples of query operations on an array of nested documents using the com.mongodb.client.MongoCollection.find method in the MongoDB Java Synchronous Driver.
Tip
The driver provides com.mongodb.client.model.Filters helper methods to facilitate the creation of filter documents. The examples on this page use these methods to create the filter documents.
The examples on this page use the inventory
collection. Connect to a
test database in your MongoDB instance then create the inventory
collection:
This page provides examples of query operations on an array of nested documents using the Collection.find() method in the MongoDB Node.js Driver.
The examples on this page use the inventory
collection. Connect to a
test database in your MongoDB instance then create the inventory
collection:
This page provides examples of query operations on an array of nested documents using the
MongoDB\Collection::find()
method in the
MongoDB PHP Library.
The examples on this page use the inventory
collection. Connect to a
test database in your MongoDB instance then create the inventory
collection:
This page provides examples of query operations on an array of nested documents using the
motor.motor_asyncio.AsyncIOMotorCollection.find()
method in the Motor
driver.
The examples on this page use the inventory
collection. Connect to a
test database in your MongoDB instance then create the inventory
collection:
This page provides examples of query operations on an array of nested documents using the com.mongodb.reactivestreams.client.MongoCollection.find method in the MongoDB Java Reactive Streams Driver.
The examples on this page use the inventory
collection. Connect to a
test database in your MongoDB instance then create the inventory
collection:
This page provides examples of query operations on an array of nested documents using the MongoCollection.Find() method in the MongoDB C# Driver.
The examples on this page use the inventory
collection. Connect to a
test database in your MongoDB instance then create the inventory
collection:
This page provides examples of query operations on an array of nested documents using the MongoDB::Collection::find() method in the MongoDB Perl Driver.
The examples on this page use the inventory
collection. Connect to a
test database in your MongoDB instance then create the inventory
collection:
This page provides examples of query operations on an array of nested documents using the Mongo::Collection#find() method in the ‘MongoDB Ruby Driver <https://docs.mongodb.com/ruby-driver/master/>`_.
The examples on this page use the inventory
collection. Connect to a
test database in your MongoDB instance then create the inventory
collection:
This page provides examples of query operations on an array of nested documents using the collection.find() method in the MongoDB Scala Driver.
The examples on this page use the inventory
collection. Connect to a
test database in your MongoDB instance then create the inventory
collection:
This page provides examples of query operations on an array of nested documents using the Collection.Find function in the MongoDB Go Driver.
The examples on this page use the inventory
collection. Connect to a
test database in your MongoDB instance then create the inventory
collection:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Query for a Document Nested in an Array¶
The following example selects all documents where an element in the
instock
array matches the specified document:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
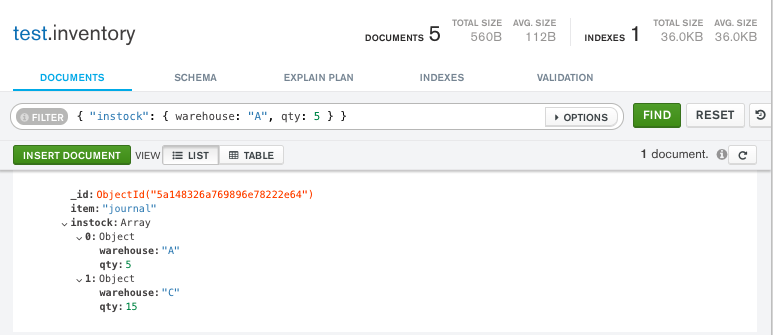
Equality matches on the whole embedded/nested document require an
exact match of the specified document, including the field order. For
example, the following query does not match any documents in the
inventory
collection:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
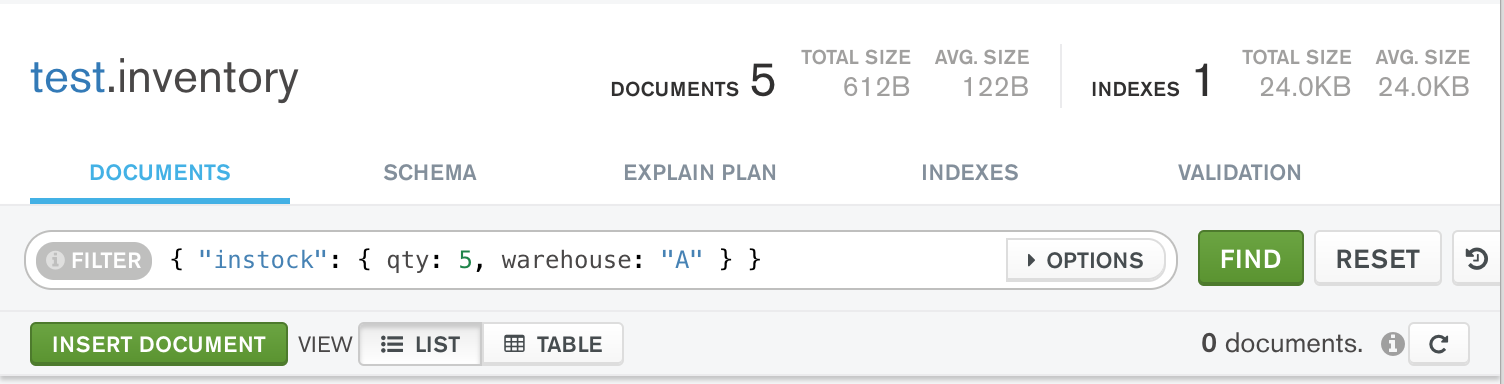
Specify a Query Condition on a Field in an Array of Documents¶
Specify a Query Condition on a Field Embedded in an Array of Documents¶
If you do not know the index position of the document nested in the
array, concatenate the name of the array field, with a dot (.
) and
the name of the field in the nested document.
The following example selects all documents where the instock
array
has at least one embedded document that contains the field qty
whose value is less than or equal to 20
:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
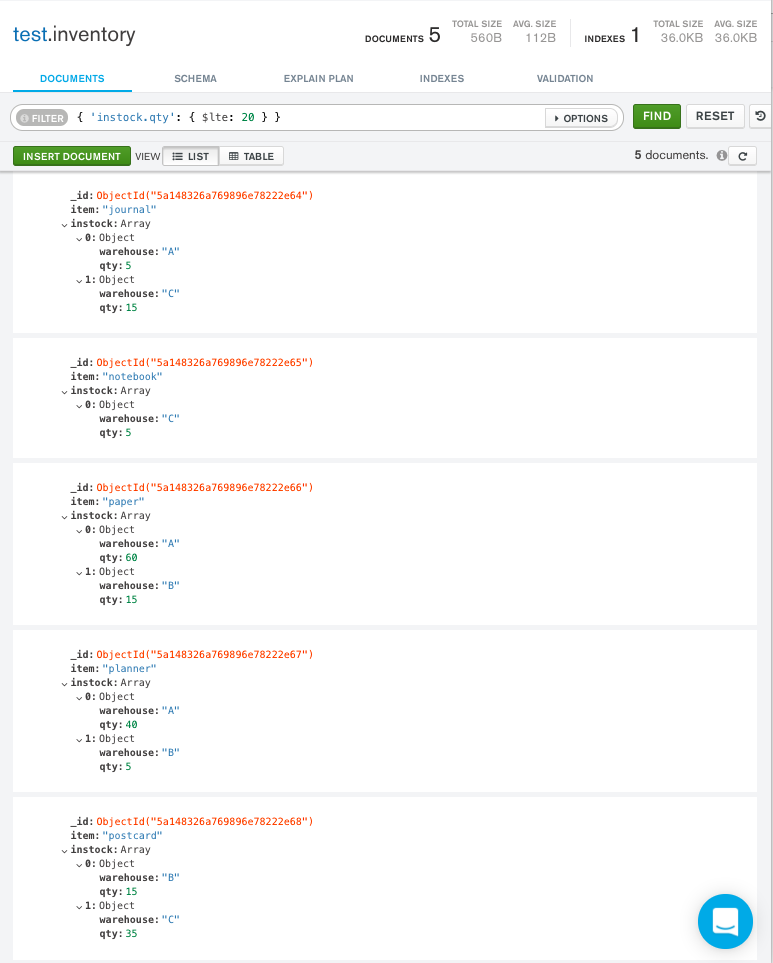
Use the Array Index to Query for a Field in the Embedded Document¶
Using dot notation, you can specify query conditions for field in a document at a particular index or position of the array. The array uses zero-based indexing.
Note
When querying using dot notation, the field and index must be inside quotation marks.
The following example selects all documents where the instock
array
has as its first element a document that contains the field qty
whose value is less than or equal to 20
:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
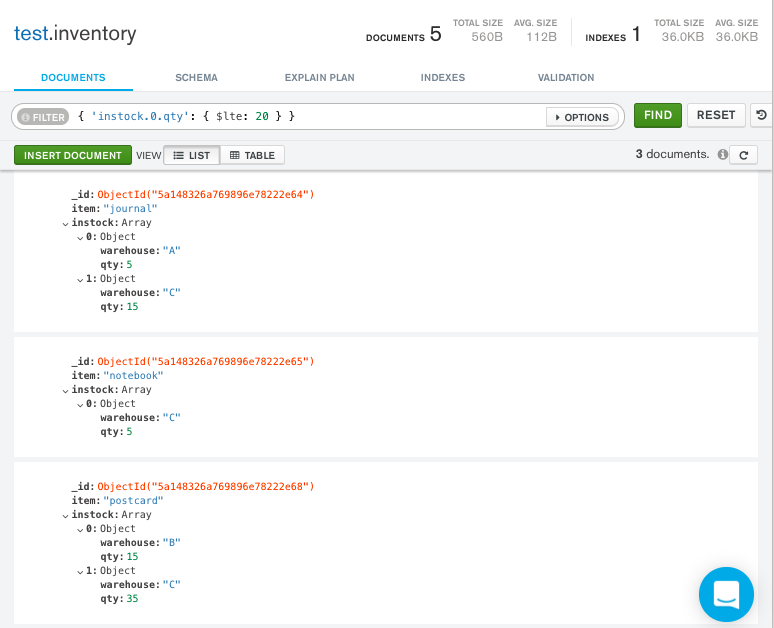
Specify Multiple Conditions for Array of Documents¶
When specifying conditions on more than one field nested in an array of documents, you can specify the query such that either a single document meets these condition or any combination of documents (including a single document) in the array meets the conditions.
A Single Nested Document Meets Multiple Query Conditions on Nested Fields¶
Use $elemMatch
operator to specify multiple criteria on an
array of embedded documents such that at least one embedded document
satisfies all the specified criteria.
The following example queries for documents where the instock
array
has at least one embedded document that contains both the field
qty
equal to 5
and the field warehouse
equal
to A
:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
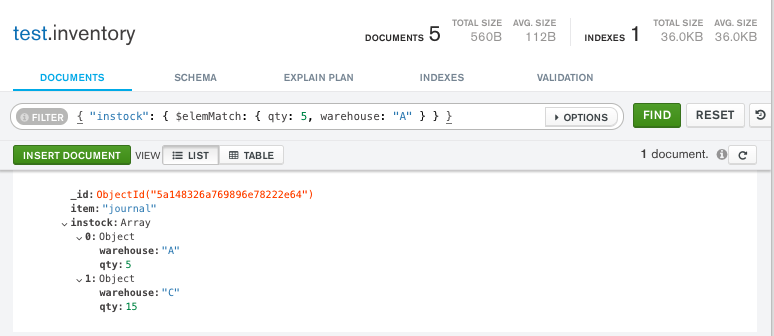
The following example queries for documents where the instock
array
has at least one embedded document that contains the field qty
that
is greater than 10
and less than or equal to 20
:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
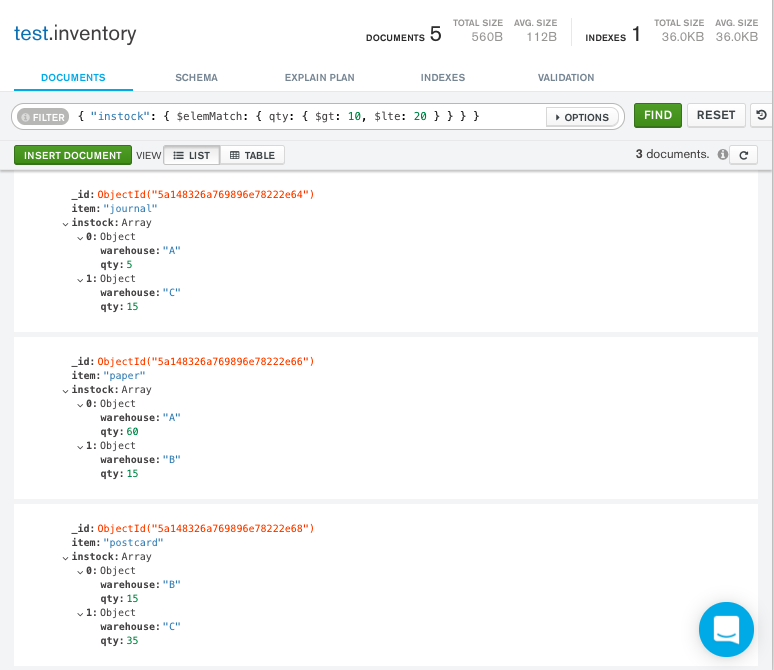
Combination of Elements Satisfies the Criteria¶
If the compound query conditions on an array field do not use the
$elemMatch
operator, the query selects those documents whose
array contains any combination of elements that satisfies the
conditions.
For example, the following query matches documents where any document
nested in the instock
array has the qty
field greater than
10
and any document (but not necessarily the same embedded
document) in the array has the qty
field less than or equal to
20
:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
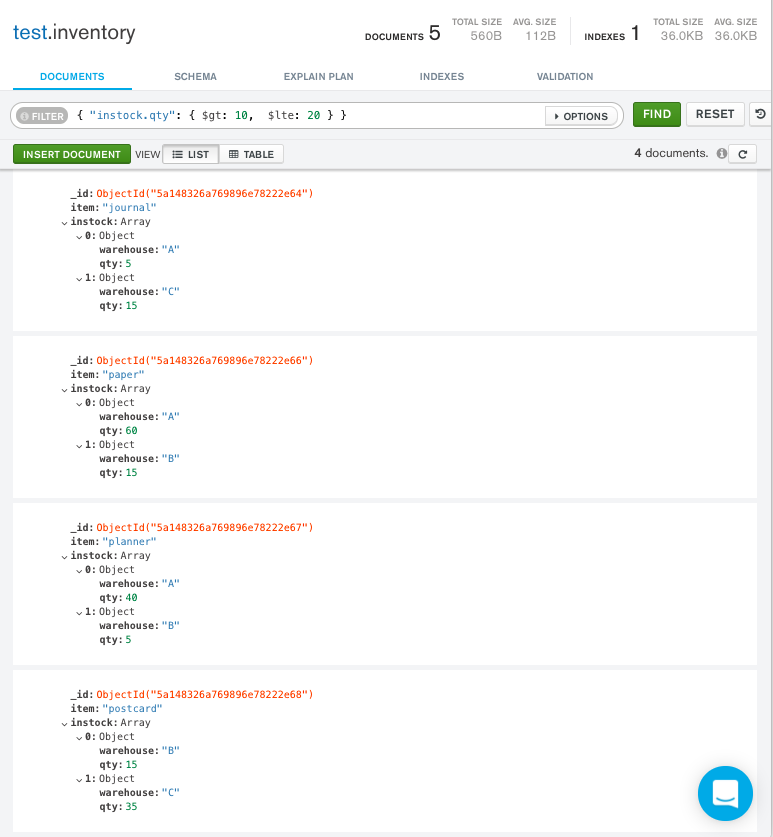
The following example queries for documents where the instock
array
has at least one embedded document that contains the field qty
equal to 5
and at least one embedded document (but not necessarily
the same embedded document) that contains the field warehouse
equal
to A
:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
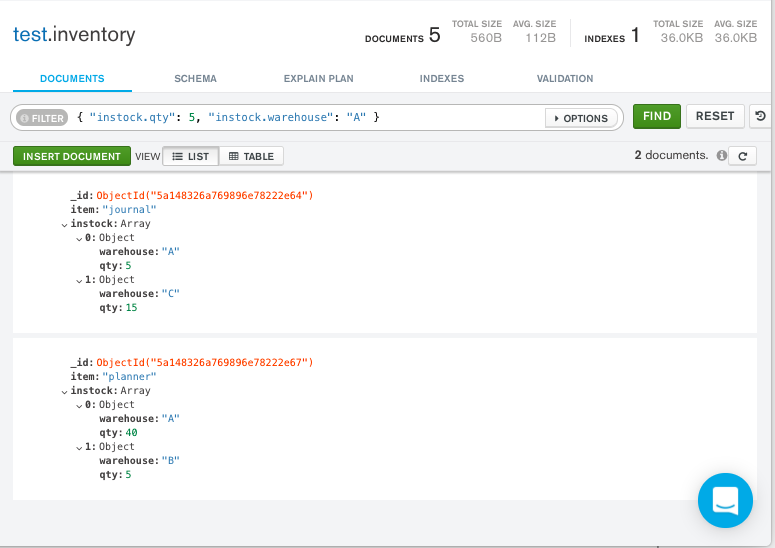
Additional Query Tutorials¶
For additional query examples, see: