- MongoDB CRUD Operations >
- Query Documents >
- Query on Embedded/Nested Documents
Query on Embedded/Nested Documents¶
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
This page provides examples of query operations on embedded/nested documents using the
db.collection.find()
method in the
mongo
shell. The examples on this page use the
inventory
collection. To populate the inventory
collection, run the following:
This page provides examples of query operations on embedded/nested documents using
MongoDB Compass. The examples on this
page use the inventory
collection. Populate the
inventory
collection with the following documents:
This page provides examples of query operations on embedded/nested documents using the
pymongo.collection.Collection.find()
method in the
PyMongo
Python driver. The examples on this page use the inventory
collection. To populate the inventory
collection, run the
following:
This page provides examples of query operations on embedded/nested documents using the com.mongodb.client.MongoCollection.find method in the MongoDB Java Synchronous Driver.
Tip
The driver provides com.mongodb.client.model.Filters helper methods to facilitate the creation of filter documents. The examples on this page use these methods to create the filter documents.
The examples on this page use the inventory
collection. To populate the inventory
collection, run the
following:
This page provides examples of query operations on embedded/nested documents using the
Collection.find() method in
the MongoDB Node.js Driver.
The examples on this page use the inventory
collection. To
populate the inventory
collection, run the following:
This page provides examples of query operations on embedded/nested documents using the
MongoDB\Collection::find()
method in the
MongoDB PHP Library.
The examples on this page use the inventory
collection. To
populate the inventory
collection, run the following:
This page provides examples of query operations on embedded/nested documents using the
motor.motor_asyncio.AsyncIOMotorCollection.find()
method in the Motor
driver. The examples on this page use the inventory
collection. To populate the inventory
collection, run the
following:
This page provides examples of query operations on embedded/nested documents using the com.mongodb.reactivestreams.client.MongoCollection.find method in the MongoDB Java Reactive Streams Driver.
The examples on this page use the inventory
collection. To populate the inventory
collection, run the
following:
This page provides examples of query operations on embedded/nested documents using the
MongoCollection.Find()
method in the
MongoDB C# Driver.
The examples on this page use the inventory
collection. To
populate the inventory
collection, run the following:
This page provides examples of query operations on embedded/nested documents using the
MongoDB::Collection::find() method
in the
MongoDB Perl Driver.
The examples on this page use the inventory
collection. To
populate the inventory
collection, run the following:
This page provides examples of query operations on embedded/nested documents using the
Mongo::Collection#find()
method in the
MongoDB Ruby Driver.
The examples on this page use the inventory
collection. To
populate the inventory
collection, run the following:
This page provides examples of query operations on embedded/nested documents using the
collection.find() method
in the
MongoDB Scala Driver.
The examples on this page use the inventory
collection. To
populate the inventory
collection, run the following:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Match an Embedded/Nested Document¶
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
To specify an equality condition on a field that is an
embedded/nested document, use the
query filter document
{ <field>: <value> }
where <value>
is the document
to match.
To specify an equality condition on a field that is an
embedded/nested document, use the
query filter document
{ <field>: <value> }
where <value>
is the document
to match.
To specify an equality condition on a field that is an
embedded/nested document, use the
query filter document
{ <field>: <value> }
where <value>
is the document
to match.
To specify an equality condition on a field that is an
embedded/nested document, use the filter document
eq( <field1>, <value>)
where <value>
is the document
to match.
To specify an equality condition on a field that is an
embedded/nested document, use the
query filter document
{ <field>: <value> }
where <value>
is the document
to match.
To specify an equality condition on a field that is an
embedded/nested document, use the
query filter document
[ <field> => <value> ]
where <value>
is the document
to match.
To specify an equality condition on a field that is an
embedded/nested document, use the
query filter document
{ <field>: <value> }
where <value>
is the document
to match.
To specify an equality condition on a field that is an
embedded/nested document, use the filter document
eq( <field1>, <value>)
where <value>
is the document
to match.
To specify an equality condition on a field that is an embedded/nested document, construct a filter using the Eq method:
<value>
is the document to match.
To specify an equality condition on a field that is an
embedded/nested document, use the
query filter document
{ <field> => <value> }
where <value>
is the document
to match.
To specify an equality condition on a field that is an
embedded/nested document, use the
query filter document
{ <field> => <value> }
where <value>
is the document
to match.
To specify an equality condition on a field that is an
embedded/nested document, use the filter document
equal( <field1>, <value> )
where <value>
is the document
to match.
For example, the following query selects all documents where the field
size
equals the document { h: 14, w: 21, uom: "cm" }
:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
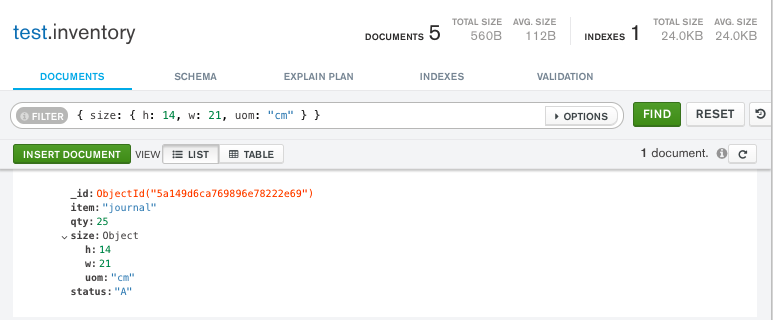
Equality matches on the whole embedded document require an exact
match of the specified <value>
document, including the field order.
For example, the following query does not match any documents in the
inventory
collection:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
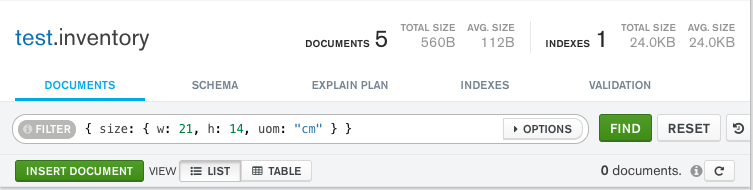
Query on Nested Field¶
To specify a query condition on fields in an embedded/nested document,
use dot notation ("field.nestedField"
).
Note
When querying using dot notation, the field and nested field must be inside quotation marks.
Specify Equality Match on a Nested Field¶
The following example selects all documents where the field uom
nested in the size
field equals "in"
:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
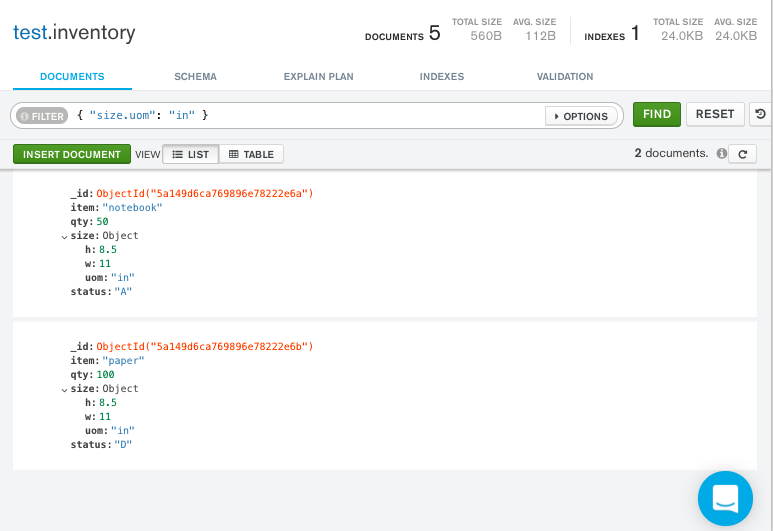
Specify Match using Query Operator¶
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
A query filter document can use the query operators to specify conditions in the following form:
A query filter document can use the query operators to specify conditions in the following form:
A query filter document can use the query operators to specify conditions in the following form:
In addition to the equality condition, MongoDB provides various query operators to specify filter conditions. Use the com.mongodb.client.model.Filters helper methods to facilitate the creation of filter documents. For example:
A query filter document can use the query operators to specify conditions in the following form:
A query filter document can use the query operators to specify conditions in the following form:
A query filter document can use the query operators to specify conditions in the following form:
In addition to the equality condition, MongoDB provides various query operators to specify filter conditions. Use the com.mongodb.client.model.Filters helper methods to facilitate the creation of filter documents. For example:
In addition to the equality filter, MongoDB provides various query operators to specify filter conditions. Use the FilterDefinitionBuilder methods to create a filter document. For example:
A query filter document can use the query operators to specify conditions in the following form:
A query filter document can use the query operators to specify conditions in the following form:
In addition to the equality condition, MongoDB provides
various query operators to specify
filter conditions. Use the
com.mongodb.client.model.Filters_
helper methods to
facilitate the creation of filter documents. For example:
The following query uses the less than operator ($lt
) on
the field h
embedded in the size
field:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
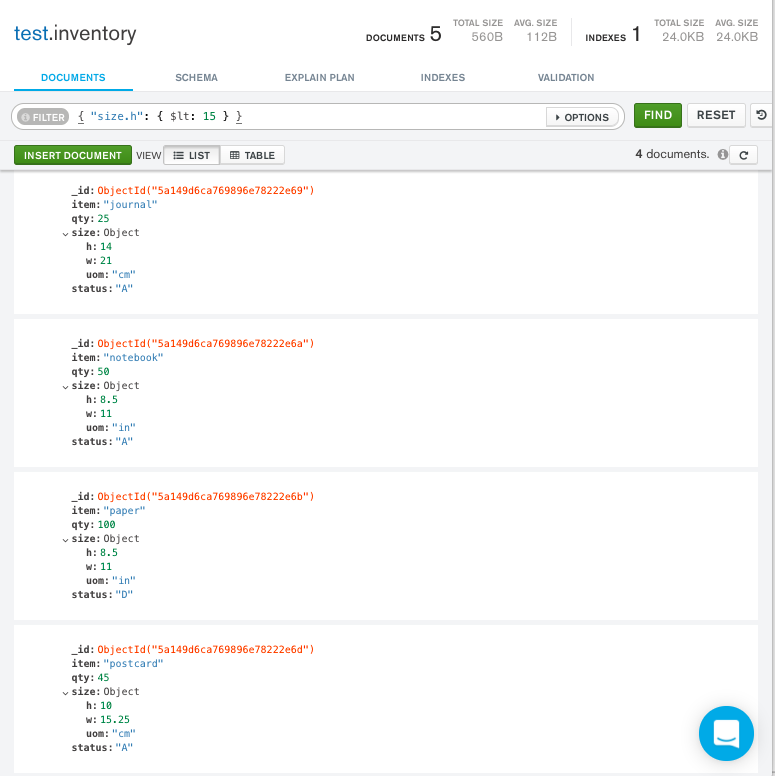
Additional Query Tutorials¶
For additional query examples, see: